AngularJS – Slugify Filter
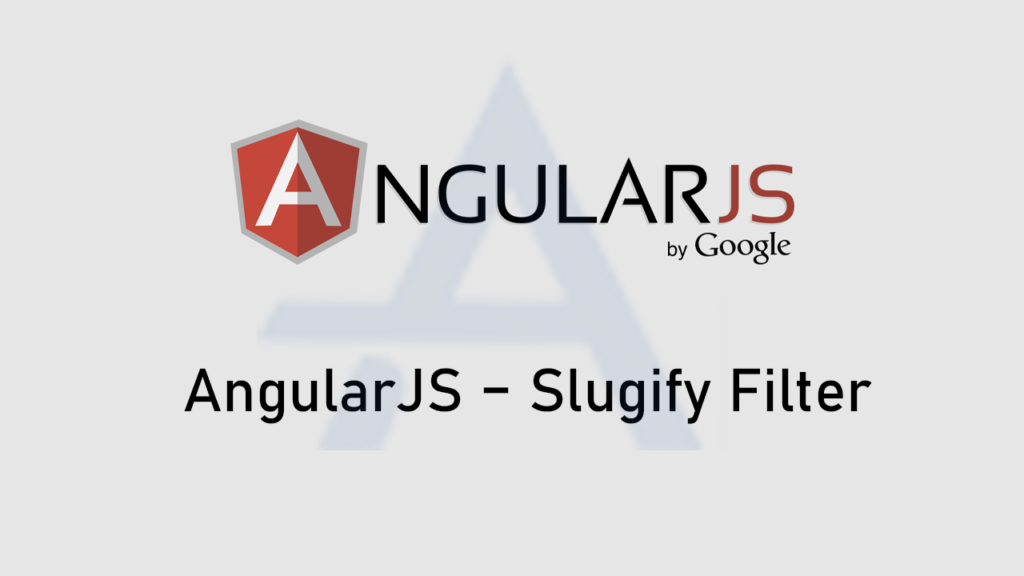
A custom AngularJS filter for converting a string into a url slug. A slug is a url & seo friendly string containing only lowercase alphanumeric characters and hyphens “-“. Slugs are often used for including titles in URLs for SEO. URL slug in angular js
app.filter('slugify', function() { return function(input) { if (!input) return ''; // Convert the string to lowercase var slug = input.toLowerCase(); // Remove special characters slug = slug.replace(/[^a-z0-9\s-]/g, ''); // Replace spaces and underscores with hyphens slug = slug.replace(/[\s_]+/g, '-'); // Remove any leading or trailing hyphens slug = slug.replace(/^-+|-+$/g, ''); return slug; }; });
Use the Slug Filter in Your Controller or View
Once you have the filter in place, you can use it in your AngularJS controller or directly in the view.
In Controller:
app.controller('MyController', function($scope, $filter) { $scope.title = "My Awesome Blog Post!"; $scope.slug = $filter('slugify')($scope.title); });
In View:
<div ng-controller="MyController"> <p>Original Title: {{ title }}</p> <p>URL Slug: {{ title | slugify }}</p> </div>
Example
If your original title is "Angular js slug creation!"
, the slug generated would be angular-js-slug-creation.
Explanation:
- Lowercase Conversion: The string is converted to lowercase for uniformity.
- Special Characters Removal: All special characters except spaces and hyphens are removed.
- Space Replacement: Spaces are replaced with hyphens to create the slug.
- Hyphen Trimming: Any leading or trailing hyphens are removed.
This method allows you to easily create SEO-friendly URL slugs within your AngularJS application.