How to Integrate PhonePe Payment Gateway in PHP
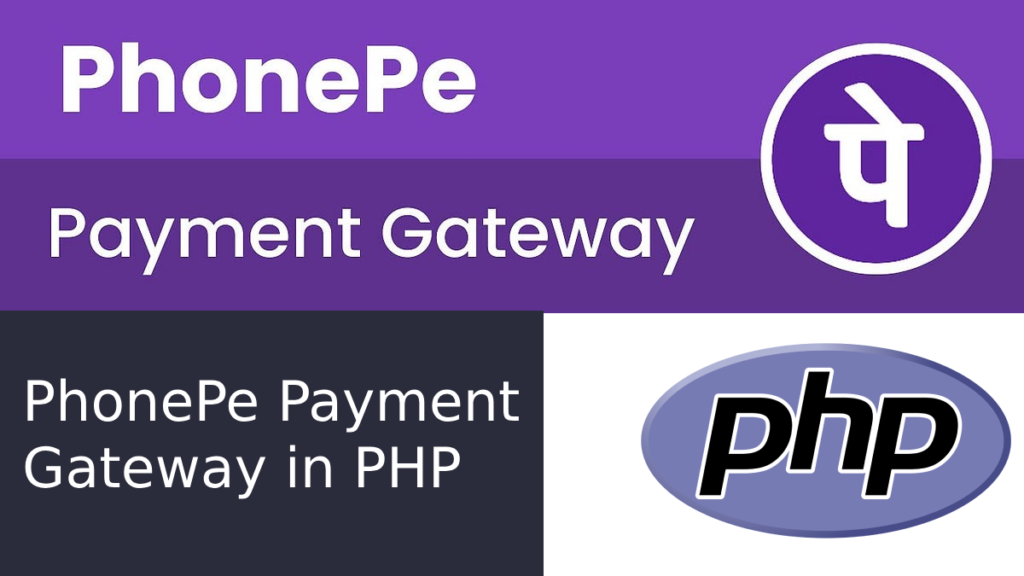
Integrating a payment gateway is a crucial step for any e-commerce website or application. It ensures smooth transactions and enhances user experience. In this guide, weโll walk you through the process of integrating the PhonePe payment gateway into a PHP application. Letโs dive in!
How to Integrate PhonePe Payment Gateway in PHP
Introduction
Integrating a payment gateway is essential for any online business, ensuring seamless transactions and a better user experience. PhonePe is one of India’s leading payment gateways, known for its reliability and ease of use. In this article, we’ll show you how to integrate the PhonePe payment gateway into a PHP application, covering every detail from setup to implementation.
Prerequisites
Before diving into the integration, make sure you have the following:
- A PhonePe Merchant Account: You need to sign up and get approval from PhonePe.
- PHP Installed: Ensure PHP is installed on your server.
- cURL Library: This is required for making HTTP requests from your PHP script.
- SSL Certificate: For secure transactions, SSL is mandatory.
Getting Started with PhonePe
Creating a PhonePe Merchant Account
First, you need to create a merchant account with PhonePe. Visit the PhonePe Merchant Portal, sign up, and complete the necessary KYC (Know Your Customer) procedures. Once your account is approved, you’ll get access to the merchant dashboard where you can manage your API keys and view transaction reports.
Understanding PhonePe API Documentation
PhonePe provides comprehensive API documentation which is essential for integration. Make sure you go through the PhonePe API documentation to understand the endpoints, request formats, and response handling.
Setting Up Your PHP Project
Installing Necessary Libraries
Ensure you have the required libraries installed. The most important one is cURL. You can check if cURL is installed by running:
php -m | grep curl
If it’s not installed, you can install it using:
sudo apt-get install php-curl
Configuring Your Project
Set up your project structure. Here’s a simple structure to get you started:
/your-project /src - PaymentHandler.php - index.php - config.php
PhonePe Integration Steps
Step 1: Generate the API Key
Log into the PhonePe Merchant Dashboard and navigate to the API Keys section. Generate a new API key and keep it secure. You’ll need this key to authenticate your requests.
Step 2: Setup Webhooks
Webhooks allow PhonePe to notify your server about the payment status. Configure your webhook URL in the PhonePe dashboard to handle payment status updates.
Step 3: Create Payment Request
Your PHP script will need to create a payment request. This involves sending a POST request to the PhonePe API with the required parameters like amount, transaction ID, and callback URL.
Step 4: Handle Payment Response
Once the payment is processed, PhonePe will send a response to your server. Your script must handle this response to update the payment status in your database.
Detailed Code Implementation
Generating the Signature
PhonePe requires all requests to be signed with a key. Here’s how you can generate the signature in PHP:
function generateSignature($apiKey, $requestBody) { return hash_hmac('sha256', $requestBody, $apiKey); }
Creating the Payment Request in PHP
Hereโs an example of how to create a payment request:
$apiKey = 'your_api_key'; $merchantId = 'your_merchant_id'; $amount = 1000; // Amount in paise $transactionId = uniqid(); $callbackUrl = 'https://yourwebsite.com/callback'; $requestBody = json_encode([ 'merchantId' => $merchantId, 'transactionId' => $transactionId, 'amount' => $amount, 'callbackUrl' => $callbackUrl, ]); $signature = generateSignature($apiKey, $requestBody); $ch = curl_init('https://api.phonepe.com/v3/transaction/initiate'); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, $requestBody); curl_setopt($ch, CURLOPT_HTTPHEADER, [ 'Content-Type: application/json', 'X-VERIFY: ' . $signature, ]); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); $responseData = json_decode($response, true); if ($responseData['success']) { echo "Payment initiated successfully!"; } else { echo "Error initiating payment: " . $responseData['message']; }
Verifying the Payment Response
Once PhonePe processes the payment, it sends a response to your callback URL. Handle this response to update the payment status.
$callbackResponse = file_get_contents('php://input'); $callbackSignature = $_SERVER['HTTP_X_VERIFY']; $computedSignature = generateSignature($apiKey, $callbackResponse); if ($computedSignature === $callbackSignature) { $response = json_decode($callbackResponse, true); // Process the response (e.g., update the database) echo "Payment status: " . $response['status']; } else { echo "Invalid signature!"; }
Handling Payment Status
Checking Payment Status
You can check the payment status using the PhonePe API. This is useful if you want to verify the payment status manually or periodically.
$transactionId = 'your_transaction_id'; $statusUrl = 'https://api.phonepe.com/v3/transaction/' . $transactionId . '/status'; $signature = generateSignature($apiKey, ''); $ch = curl_init($statusUrl); curl_setopt($ch, CURLOPT_HTTPHEADER, [ 'X-VERIFY: ' . $signature, ]); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); $responseData = json_decode($response, true); if ($responseData['success']) { echo "Payment status: " . $responseData['status']; } else { echo "Error fetching payment status: " . $responseData['message']; }
Managing Payment Success and Failures
Ensure your application properly handles both successful and failed payments by updating the transaction status in your database and notifying the user.
Security Considerations
Secure API Communication
Always use HTTPS for secure communication with the PhonePe API. Ensure your server has a valid SSL certificate.
Data Validation and Sanitization
Validate and sanitize all inputs and outputs to prevent security vulnerabilities like SQL injection and cross-site scripting (XSS).
Testing the Integration
Testing in Sandbox Mode
PhonePe provides a sandbox environment for testing. Use it to ensure your integration works correctly before going live.
Moving to Production
Once testing is successful, switch to the production environment by updating the API endpoints and keys.
Troubleshooting Common Issues
Common Errors and Fixes
- Invalid Signature: Ensure your signature generation logic matches PhonePe’s requirements.
- Network Issues: Check your server’s internet connection and firewall settings.
PhonePe Support and Resources
If you encounter issues, refer to the PhonePe developer resources or contact their support for assistance.
Enhancing User Experience
- Customizing Payment Pages: Customize the payment pages to match your websiteโs theme for a seamless user experience.
- Providing Real-time Feedback: Inform users about payment status in real-time using webhooks and dynamic UI updates.
Maintaining Your Integration
- Regular Updates and Maintenance: Keep your integration updated with the latest API changes and security patches.
- Monitoring and Analytics: Monitor transaction logs and use analytics to track payment performance and identify issues.
Advanced Tips
- Optimizing Performance: Optimize your code and server configuration to handle high transaction volumes efficiently.
- Scaling for High Traffic: Ensure your infrastructure can scale to accommodate peak traffic and high transaction loads.
Conclusion
Integrating the PhonePe payment gateway into your PHP application involves several steps, from setting up your merchant account to handling payment responses. By following this guide, you can ensure a smooth and secure payment process for your users, enhancing their overall experience and boosting your business’s credibility.
FAQs
Is there a limit to the number of transactions?
PhonePe does not impose a specific limit on the number of transactions, but high transaction volumes should be discussed with their support team for optimal performance.
How do I get support from PhonePe?
Visit the PhonePe Merchant Support section on their website or contact their customer service for assistance.
What should I do if a payment fails?
Handle payment failures gracefully by notifying the user and providing alternative payment options or retry mechanisms.
Can I integrate PhonePe with other payment gateways?
Yes, you can integrate PhonePe alongside other payment gateways to offer your users multiple payment options.
How secure is PhonePe?
PhonePe uses advanced encryption and secure protocols to ensure the safety of transactions, making it a highly secure payment gateway.