How to make Email Verifications in Laravel
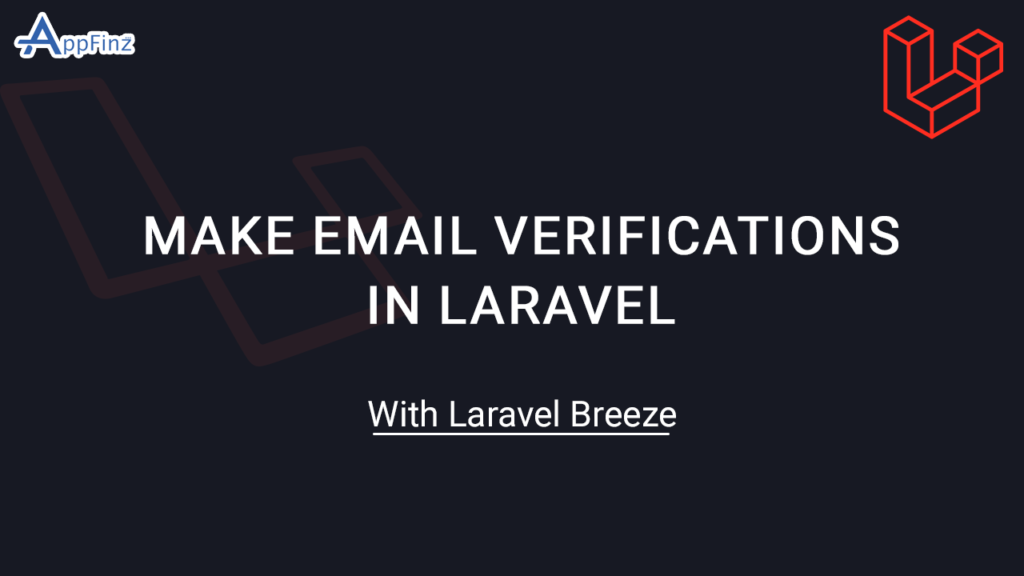
Hello Artisans, In this blog we will see how to implement Email Verifications in Laravel, How Email Verification works with Laravel Application, We will use laravel Breeze package to implement Email Verifications in Laravel Application, To implement email verification in Laravel, follow these steps:
Step 1: Set Up Your Laravel Project
If you don’t have a Laravel project yet, create one:
composer create-project --prefer-dist laravel/laravel laravel-email-verification
Step 2: Set Up Authentication
Install Laravel Breeze or Laravel Jetstream for authentication scaffolding. Here, we’ll use Laravel Breeze:
composer require laravel/breeze --dev php artisan breeze:install php artisan migrate npm install && npm run dev
Step 3: Configure Email Settings
Set up your email configuration in the .env
file. Add your SMTP server details:
MAIL_MAILER=smtp MAIL_HOST=smtp.mailtrap.io MAIL_PORT=2525 MAIL_USERNAME=your_username MAIL_PASSWORD=your_password MAIL_ENCRYPTION=tls MAIL_FROM_ADDRESS=noreply@example.com MAIL_FROM_NAME="${APP_NAME}"
Step 4: Enable Email Verification
In your User
model (app/Models/User.php
), make sure the MustVerifyEmail
interface is implemented:
use Illuminate\Contracts\Auth\MustVerifyEmail; class User extends Authenticatable implements MustVerifyEmail { // other model methods and properties }
Step 5: Update Auth Routes
In routes/web.php
, add the following route to handle email verification:
use Illuminate\Foundation\Auth\EmailVerificationRequest; use Illuminate\Http\Request; Route::get('/email/verify', function () { return view('auth.verify-email'); })->middleware('auth')->name('verification.notice'); Route::get('/email/verify/{id}/{hash}', function (EmailVerificationRequest $request) { $request->fulfill(); return redirect('/home'); })->middleware(['auth', 'signed'])->name('verification.verify'); Route::post('/email/verification-notification', function (Request $request) { $request->user()->sendEmailVerificationNotification(); return back()->with('message', 'Verification link sent!'); })->middleware(['auth', 'throttle:6,1'])->name('verification.send');
Step 6: Create Email Verification View
Create a new view file for the email verification notice at resources/views/auth/verify-email.blade.php
:
@extends('layouts.app') @section('content') <div> @if (session('message')) <div> {{ session('message') }} </div> @endif <div> Before proceeding, please check your email for a verification link. If you did not receive the email, click the button below to request another. </div> <form method="POST" action="{{ route('verification.send') }}"> @csrf <button type="submit">Resend Verification Email</button> </form> </div> @endsection
Step 7: Modify Registration Controller
Make sure your registration controller sends a verification email after a user registers. In the RegisteredUserController
(or wherever your registration logic is):
use Illuminate\Auth\Events\Registered; public function store(Request $request) { // validate and create user $user = User::create([ 'name' => $request->name, 'email' => $request->email, 'password' => Hash::make($request->password), ]); event(new Registered($user)); Auth::login($user); return redirect()->route('verification.notice'); }
Step 8: Protect Routes
Ensure that your application routes are protected and only accessible to verified users. Use the verified
middleware in routes/web.php
:
Route::get('/home', [HomeController::class, 'index'])->middleware(['auth', 'verified'])->name('home');
Step 9: Test Email Verification
- Register a new user through your registration form.
- Check the registered user’s email for the verification link.
- Click the verification link to verify the email.
- Ensure the user is redirected to the intended page after verification.
I hope this blog will help you to build Make Email Verifications in Laravel