Laravel Admin Middleware for Auth Admin Users Roles [Laravel 12, Updated 2025]
Laravel Admin Pannel Setup, Laravel Middleware Updated Laravel 11
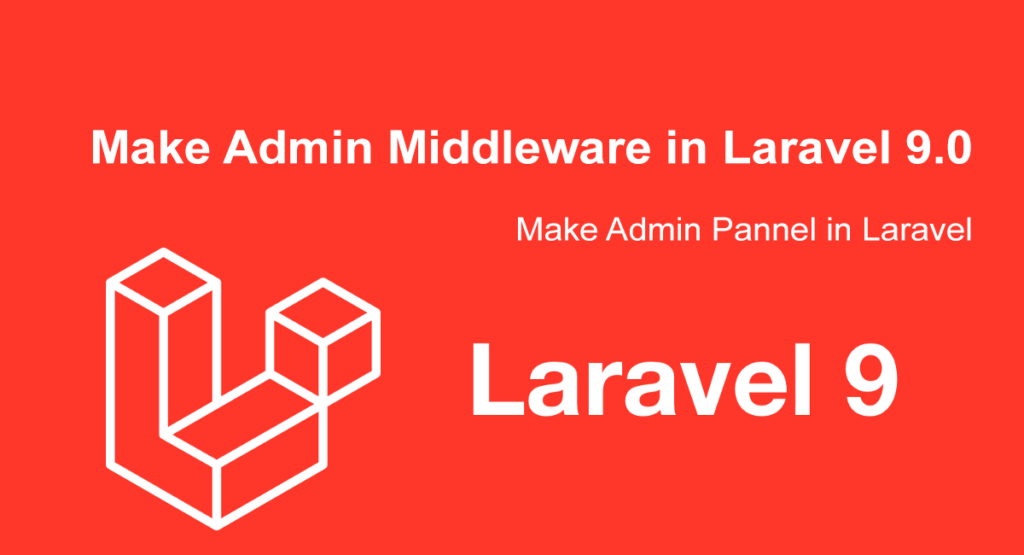
In this post, we will see How to make Admin panel working in Laravel 12 | Admin Middleware in Laravel Working
Examples of Laravel Middleware for Auth Admin Users Roles. This tutorial shows how to build a sample Laravel middleware for the admin role from scratch. Check if the authenticated user is an admin or a user. If he’s a user, he can’t get admin access to get admin pages.
Middleware provides a convenient mechanism for filtering incoming HTTP requests to your application. Laravel provides authentication middleware that validates that users of your application have been authenticated. Here we use an admin middleware where the user is not an admin and the middleware redirects the user to the dashboard.
However, if the user is an administrator, the middleware allows the request to proceed further to the application. This tutorial will show you step by step how to use middleware in Laravel, or how to call middleware in your controller. Let’s start with the single-role or multi-role Laravel middleware admin role
Laravel 11 Middleware Example Tutorial [Updated Laravel 12]
Follow the following steps to create Laravel middleware for auth admin and user roles:
- Step 1 โ Install Laravel App
- Step 2 โ Connect Database to App
- Step 3 โ Generate Laravel Authentication
- Step 4 โ Update User’s Migration
- Step 5 โ Create Middleware
- Step 6 โ Admin Protected Middleware Route
- Step 7 โ Create & Update Blade Files
- Step 8 โ Update Controller Methods
- Step 9 โ Multiple Middlewares in Single Route
Step 1: Install Laravel App
First at all, install Laravel 11 using Composer. Open a new command-line interface and run the following command:
composer create-project --prefer-dist laravel/laravel laravel-admin
Go inside the app:
cd laravel-admin
Step 2: Connect Database to App
Now, open your laravel application .env file and make the following database related changes in it.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_admin DB_USERNAME=root DB_PASSWORD=
Step 3: Generate Laravel Authentication Using Latest Laravel UI 4.x for Laravel 9, 10, 11, Here we are using Bootstrap Auth You can also use Vue or React Auth According to your need
composer require laravel/ui php artisan ui bootstrap --auth npm install npm run dev // Press CTRL + C Then Again hit below mentioned command npm run build
Open users table migration and update the is_admin field on it. Here using this is_admin we will filter our Admin user to enter admin routes
database/migrations/0001_01_01_000000_create_users_table.php
Step 4: Update User’s Migration
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. */ public function up(): void { Schema::create('users', function (Blueprint $table) { $table->bigIncrements('id'); $table->string('name'); $table->string('email')->unique(); $table->timestamp('email_verified_at')->nullable(); $table->string('password'); $table->boolean('is_admin')->nullable(); $table->rememberToken(); $table->timestamps(); }); Schema::create('password_reset_tokens', function (Blueprint $table) { $table->string('email')->primary(); $table->string('token'); $table->timestamp('created_at')->nullable(); }); Schema::create('sessions', function (Blueprint $table) { $table->string('id')->primary(); $table->foreignId('user_id')->nullable()->index(); $table->string('ip_address', 45)->nullable(); $table->text('user_agent')->nullable(); $table->longText('payload'); $table->integer('last_activity')->index(); }); } /** * Reverse the migrations. */ public function down(): void { Schema::dropIfExists('users'); Schema::dropIfExists('password_reset_tokens'); Schema::dropIfExists('sessions'); } };
After adding the column now run the migration just running the following command.
php artisan migrate
Step 5: Create Middleware
Now Create middleware for handling auth admin roles. Open the terminal and run below command.
php artisan make:middleware IsAdmin
Now you check you have generated a file in your project middleware directory, open and update the below code on it.
app\Http\Middleware\IsAdmin.php
<?php namespace App\Http\Middleware; use Closure; use Auth; class IsAdmin { /** * Handle an incoming request. * * @param \Illuminate\Http\Request $request * @param \Closure $next * @return mixed */ public function handle($request, Closure $next) { if (Auth::user() && Auth::user()->is_admin == 1) { return $next($request); } return redirect('home')->with('error','You have not admin access'); // It will redirect user back to home screen if they do not have is_admin=1 assigned in database } }
In Laravel 11, You can add/register middleware in bootstrap/app.php
->withMiddleware(function (Middleware $middleware) { $middleware->alias([ 'admin' => \App\Http\Middleware\AdminMiddleware::class, ]); })
Below Laravel 11 Application like in 8,9 or 10 laravel version, You can add/register middleware in app\Http\Kernel.php
For Laravel 8,9 or 10 open your kernel.php file and go to the protected $routeMiddleware property and update theย adminย middleware here.
app\Http\Kernel.php
protected $routeMiddleware = [ 'throttle' => \Illuminate\Routing\Middleware\ThrottleRequests::class, 'verified' => \Illuminate\Auth\Middleware\EnsureEmailIsVerified::class, 'admin' => \App\Http\Middleware\IsAdmin::class, ];
Step 6: Admin Protected Middleware Route
Create routes that an administrator must protect. If the user is not an admin, they will be redirected to the home page. Otherwise, he can visit this page. Now if I want to assign routes to this middleware admin, these routes will be secured and only accessible if the authorized user is an admin. Otherwise, you will be redirected to the home page.
php artisan make:controller AdminController
app/routes/web.php
use Illuminate\Support\Facades\Route; use App\Http\Controllers\HomeController; Route::get('/', function () { return view('welcome'); }); Auth::routes(); Route::get('/home', [App\Http\Controllers\HomeController::class, 'index'])->name('home'); Route::group(['middleware' => ['auth', 'admin']], function () { Route::get('admin-home', [App\Http\Controllers\AdminController::class, 'adminHome'])->name('admin.home'); }); // Here it is checking two middlewares auth and admin, it means if user is authenticated and having admin right (is_admin=1) only in that case they will reach this route
Step 7: Create & Update Blade Files
Add accessing page link add in the home page which is open after user login.
resources\views\home.blade.php
@extends('layouts.app') @section('content') <div class="container"> <div class="row justify-content-center"> <div class="col-md-8"> <div class="card"> <div class="card-header">Dashboard</div> <div class="card-body"> @if (session('error')) <div class="alert alert-danger"> {{ session('error') }} </div> @endif @if (session('status')) <div class="alert alert-success" role="alert"> {{ session('status') }} </div> @endif You are logged in! </div> <div class="card-body"> <div class="panel-body"> Check admin view: <a href="{{route('admin.home')}}">Admin Home</a> </div> </div> </div> </div> </div> </div> @endsection
Create a blade file if the user is authenticated with admin then access this page.
resources\views\admin-home.blade.php
@extends('layouts.app') @section('content') <div class="container"> <div class="row justify-content-center"> <div class="col-md-8"> <div class="card"> <div class="card-header">Admin Home</div> <div class="card-body"> Welcome to admin dashboard </div> </div> </div> </div> </div> @endsection
Step 8: Update Controller Methods
Now add a method in your controller if a user is authenticated with admin then admin-home function work.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class HomeController extends Controller { /** * Create a new controller instance. * * @return void */ public function __construct() { $this->middleware('auth'); } /** * Show the application dashboard. * * @return \Illuminate\Contracts\Support\Renderable */ public function index() { return view('home'); } public function adminHome() { return view('admin-home'); } }
Register Middleware in bootstrap/app.php
In Laravel 12, you register custom middleware here. Add under the middleware section:
Open: bootstrap/app.php
โ
How to Properly Register Your IsAdmin
Middleware in Laravel 12 [Updated]
So the relevant part of bootstrap/app.php
looks like:
->withMiddleware(function (Middleware $middleware) { $middleware->alias([ 'admin' => \App\Http\Middleware\IsAdmin::class, ]); })
In your laravel Application put your user table value to is_admin value to 1 for changing default user to admin access like i did open your phpmyadmin and update 1
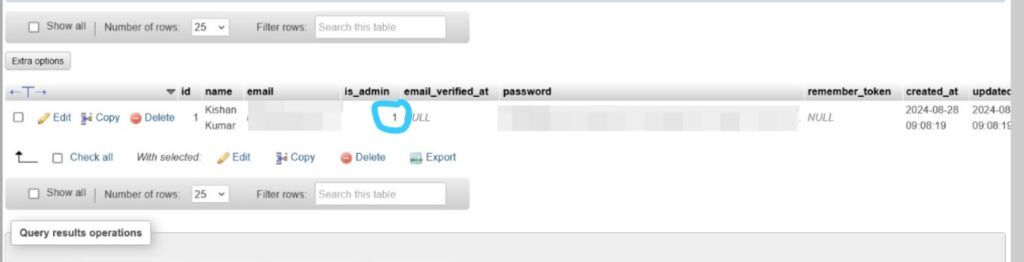
And now if you are accessing
http://localhost/laravel-admin/admin/home
Then you ll see that laravel Admin can be accessible via this url
Note: User must be registered and updated is_admin=1 is database, you can manually update it also, you can also specify different. admin routes and admin directories also.
Thanks for now, if you have any types of queries, do message me once, i ll try to help you. Happy Coding