Vue js: Access global data in template
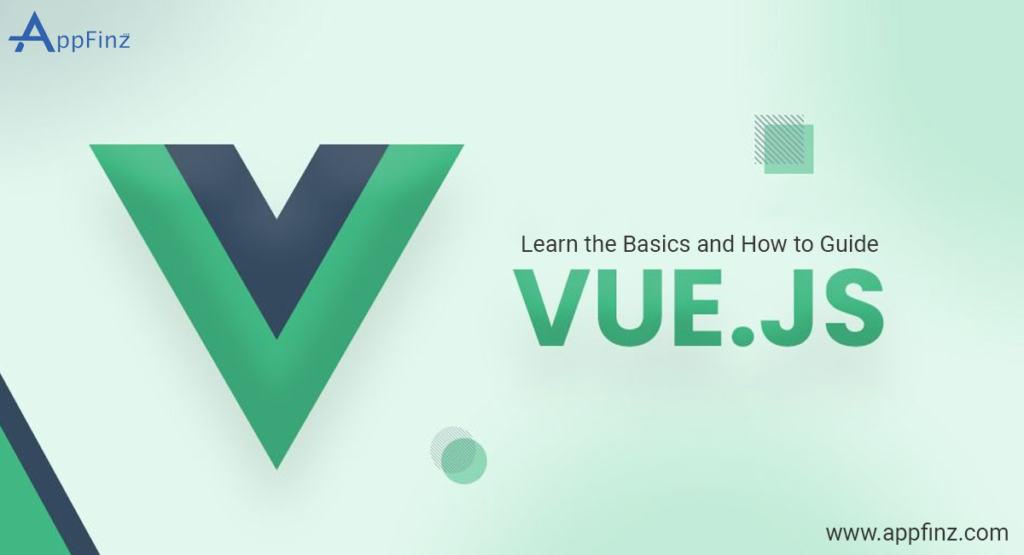
In Vue.js, you can access global data in your templates by using a few different strategies. The most common approaches include using a global event bus, Vuex for state management, or providing data through the root Vue instance. Hereโs how you can achieve this:
Using the Root Vue Instance
You can attach global data to the root Vue instance and access it in any component.
Define global data in the root instance:
new Vue({ el: '#app', data: { globalMessage: 'Hello from the root instance!' } });
Access the global data in a component:
<template> <div> <p>{{ $root.globalMessage }}</p> </div> </template> <script> export default { name: 'MyComponent' }; </script>
Using Vuex for State Management
Vuex is a state management library for Vue.js applications. Itโs a more scalable and structured way to manage global state.
Install Vuex:
npm install vuex
Set up Vuex store:
// store.js import Vue from 'vue'; import Vuex from 'vuex'; Vue.use(Vuex); export default new Vuex.Store({ state: { globalMessage: 'Hello from Vuex store!' } });
Use the Vuex store in your Vue instance:
// main.js import Vue from 'vue'; import App from './App.vue'; import store from './store'; new Vue({ el: '#app', store, render: h => h(App) });
Access the global data in a component:
<template> <div> <p>{{ globalMessage }}</p> </div> </template> <script> export default { name: 'MyComponent', computed: { globalMessage() { return this.$store.state.globalMessage; } } }; </script>
Using Provide/Inject
The provide/inject pattern allows you to share data between a parent component and its descendants without passing props through each intermediate component.
Provide data in the parent component:
<template> <div> <MyComponent /> </div> </template> <script> import MyComponent from './MyComponent.vue'; export default { name: 'ParentComponent', components: { MyComponent }, provide() { return { globalMessage: 'Hello from parent component!' }; } }; </script>
Inject data in the child component:
<template> <div> <p>{{ globalMessage }}</p> </div> </template> <script> export default { name: 'MyComponent', inject: ['globalMessage'] }; </script>