What’s the differences between PUT and PATCH? in laravel
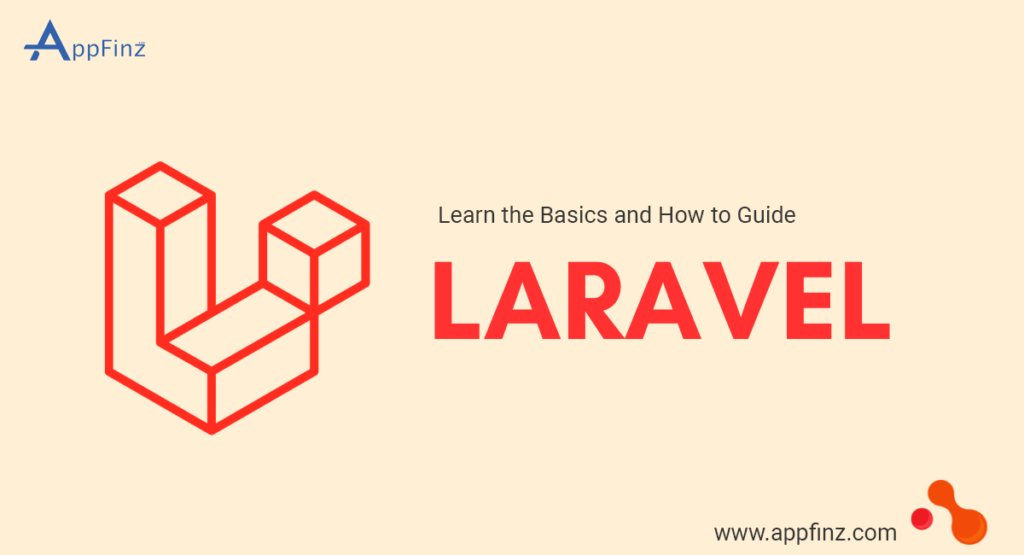
In Laravel, as in HTTP standards, both PUT
and PATCH
methods are used to update resources on the server. However, they are used differently based on the nature and scope of the update you want to perform. Hereโs a detailed explanation of the differences between PUT
and PATCH
in Laravel:
PUT Method
- Full Update: The
PUT
method is used to perform a full update of a resource. This means that the entire resource is replaced with the new data sent in the request. If any fields are omitted, they will typically be set tonull
or to their default values. - Idempotent:
PUT
requests are idempotent, meaning that making multiple identicalPUT
requests will result in the same resource state as making a single request. This ensures consistency regardless of how many times the request is made. - Request Format: When using
PUT
, you generally send all the fields of the resource, even if only some of them are being updated. - Usage in Laravel: In Laravel, you can define a
PUT
route and a corresponding controller method to handle full updates.
// routes/web.php Route::put('/resource/{id}', 'ResourceController@update'); // app/Http/Controllers/ResourceController.php public function update(Request $request, $id) { $resource = Resource::find($id); $resource->update($request->all()); return response()->json($resource); }
PATCH Method
- Partial Update: The
PATCH
method is used for partial updates to a resource. This means you only send the fields that you want to update, and the rest of the resource remains unchanged. - Not Necessarily Idempotent:
PATCH
requests are not necessarily idempotent, as subsequent requests with the same data may result in different states if the update depends on the current state of the resource. - Request Format: When using
PATCH
, you send only the fields that need to be updated. This can be more efficient for updates involving a small subset of the resourceโs attributes. - Usage in Laravel: In Laravel, you can define a
PATCH
route and a corresponding controller method to handle partial updates.
// routes/web.php Route::patch('/resource/{id}', 'ResourceController@partialUpdate'); // app/Http/Controllers/ResourceController.php public function partialUpdate(Request $request, $id) { $resource = Resource::find($id); $resource->update($request->only(['field1', 'field2'])); return response()->json($resource); }
Consider a resource representing a user profile with fields like name
, email
, and password
.
- Using
PUT
: You would send a request with all fields, even if only updating the email.
{ "name": "John Doe", "email": "john.doe@example.com", "password": "newpassword" }
Using PATCH
: You would send only the fields that need updating, such as the email.
{ "email": "john.doe@example.com" }
the differences between PUT
and PATCH
helps you choose the appropriate method based on whether you need to fully or partially update a resource.
Also Read:
Could not open input file: artisan
solve 500 internal server error in laravel
Laravel Eloquent WhereIn Query Example
Paypal Payment Gateway Integration in Laravel 11